Tutorial: Web Request
An introduction to Web APIs
Much of our day to day digital lives are powered by Web APIs. They provide the infrastructure that allows most web services and apps to work. In essence, they provide a way for an app or service to access the features provided by a server.
For example: When you use Appli to save a database record to the cloud, Appli makes a Web API request to our cloud servers to store the data.
Most Web APIs are powered by the same technologies that make the web work. They are accessible via URLs and the HTTP protocol. The most common format used to exchange data with a server is called JSON.
JSON might seem strange if you never seen it before, but it is an easy format for computers to understand. Our sample app will pick the current day and month, make a request to that API, and then show the results to the user.
In a nutshell, a very reductive nutshell, what happens is that the developer packs some instructions into a network request to a server and the server replies with the answers to those instructions. On public facing APIs, most of the features that are exposed to developers are querying features, things that make it easier to get data from a database.
Open Library API
The Open Library API is an API provided by The Internet Archive to search their extensive catalog of media. You can think of it as a massive library catalog encompassing books, games, and other media types you could find in a library. The API is divided into different sets depending on what features you need for your app. In our case we’ll use their Search API to get metadata about books (and other media) depending on search terms provided by the user.
Our sample app
Instead of creating a full feature library browsing app, something that is doable but beyond the scope of a simple tutorial on Web Requests, we’ll create a very simple app. A search box for the user to fill in with their query terms, and a display that will show the first match returned from the Search API. The API can (and will) return thousands of matches for a given search. For the sake of simplicity we’ll just show one.
Creating the UI
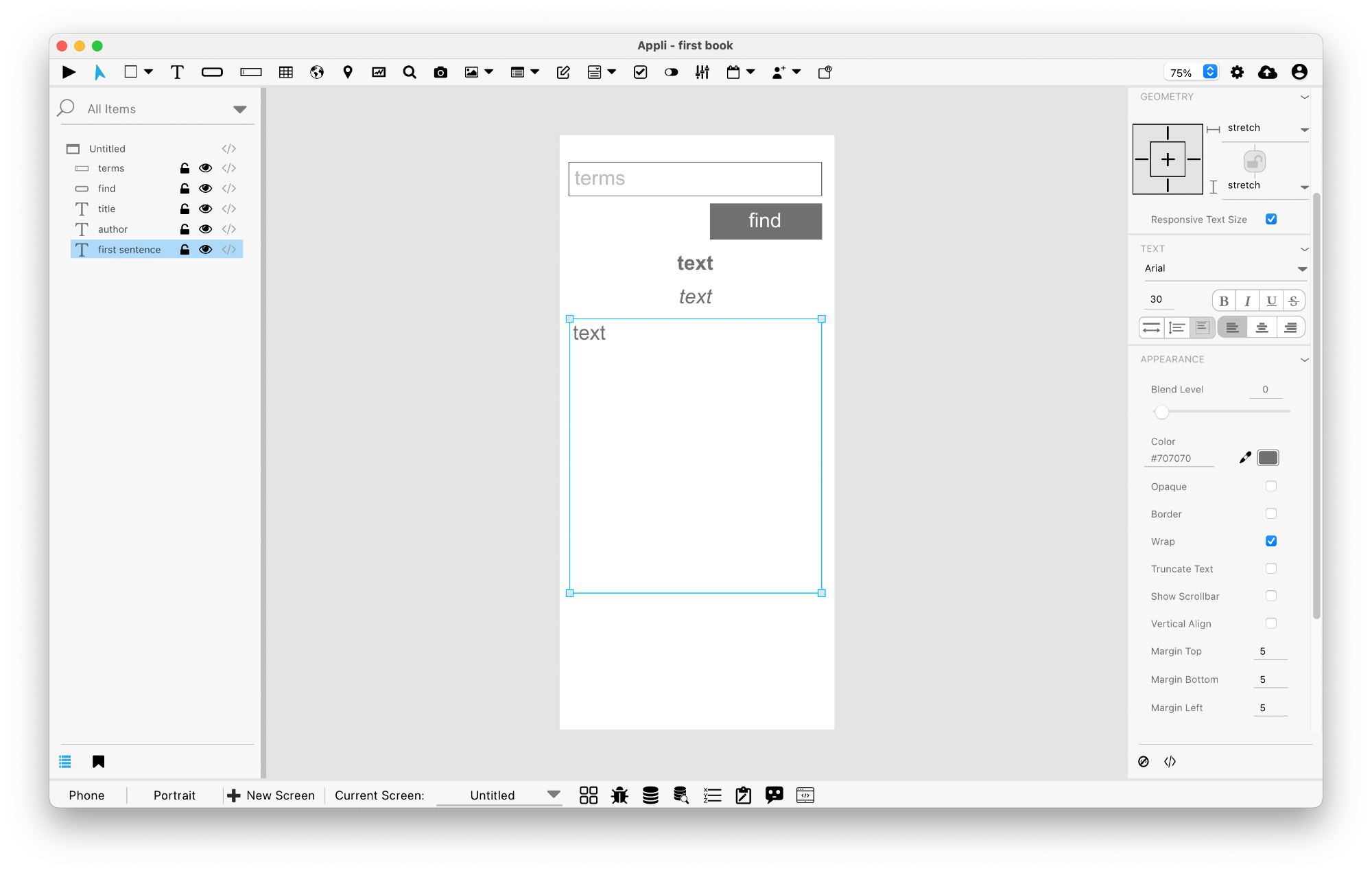
The UI consists of:
- A field element named terms.
- A button element named find.
- Three text elements named title for the book title, author for the book author, and first sentence to display the first sentence in the book.
Notice that you need to tweak the first sentence appearance to display multiple lines and wrap the text (the properties are shown in the screenshot).
Implementing the find book button
The script we’ll use for the find book button will display a loading dialog so the user knows the application is busy doing the request, make the web request and extract the data we need from the API response, and finally fill the text elements with the data and hide the loading dialog.
The search API works with URL
parameters. URL parameters (also known as query strings)
are a way to pass key and value pairs in a Web Address. The format for a
key and value pair is <key>=<value>
and each
pair is separated from the others with an ampersand. The address part is
separated from the parameters part using a question mark. So for
searching the Internet Archive catalog, for The Lord Of The
Rings, we’d make a request like for:
https://openlibrary.org/search.json?title=the+lord+of+the+rings
Notice that spaces in parameters are replaced with plus signs. If you open that URL in your browser, you’ll see the JSON response containing all the results for that query.
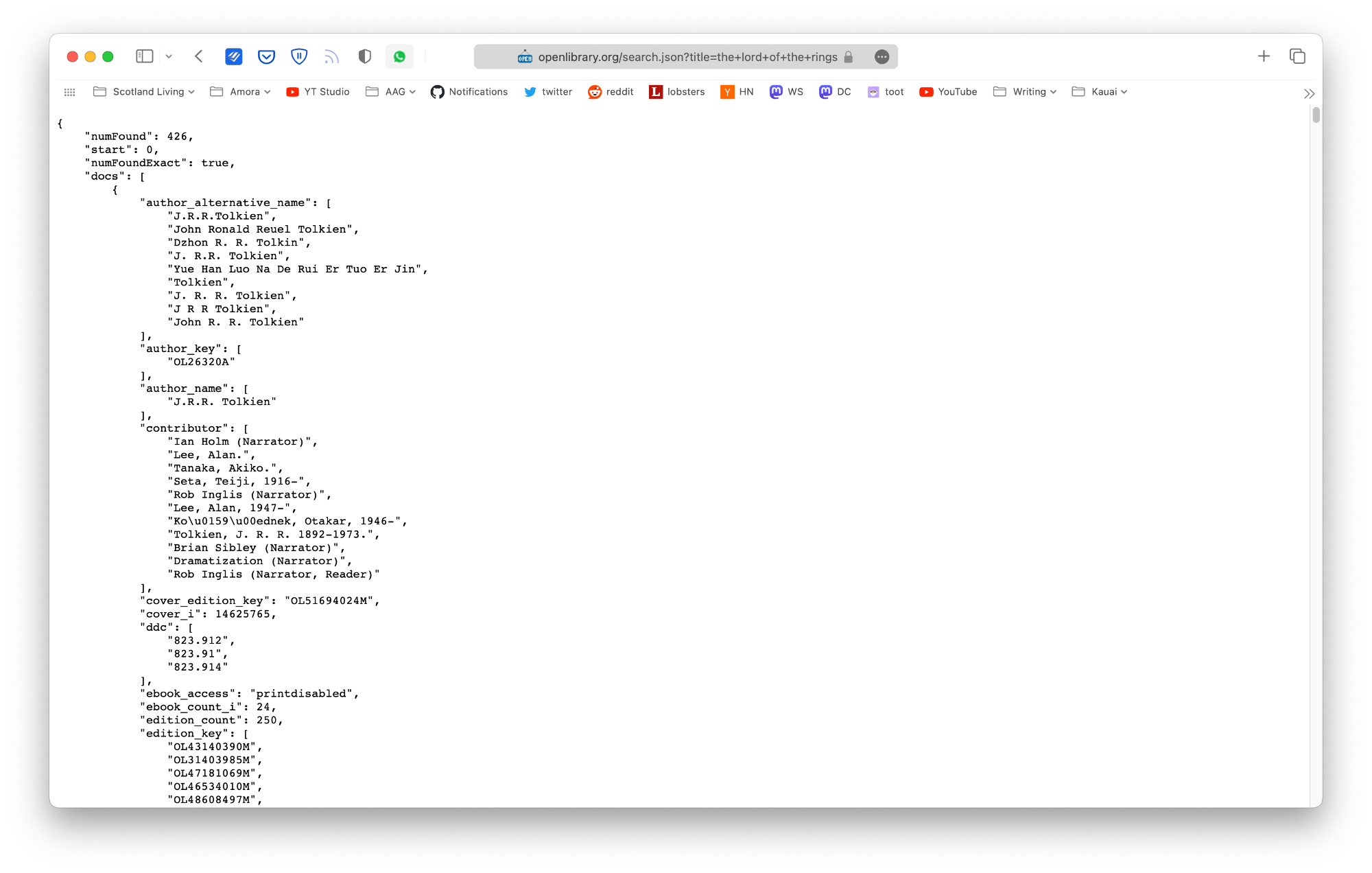
Before creating the full script for the find button, let’s create a partial version just to show how the same search results will appear inside Appli. Add this script to your find button:
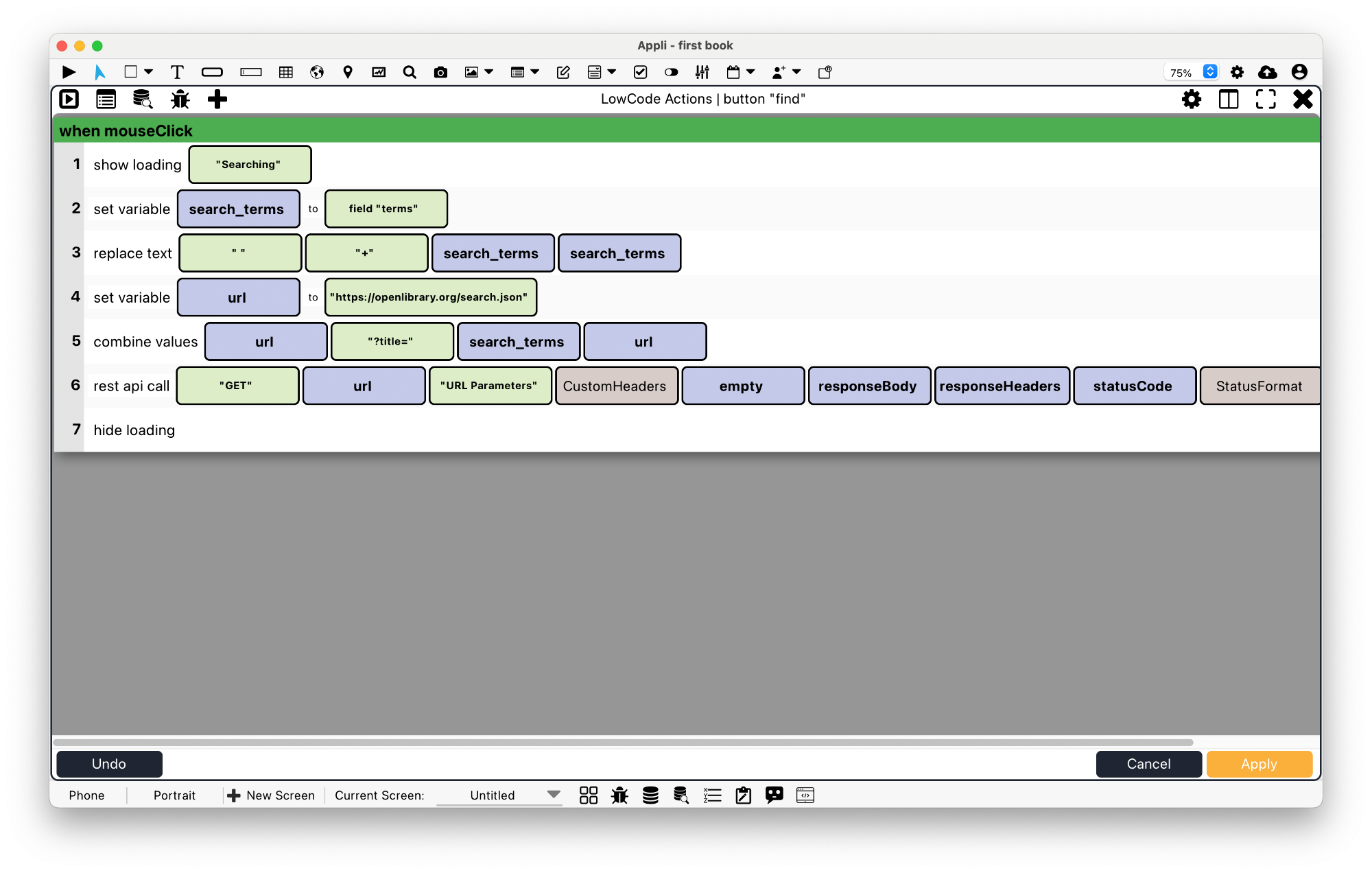
That script will execute the search and store the response in a variable called responseBody. If you switch into play mode and type “the lord of the rings” and press the find button, the app will execute the search and stop. Using the variable viewer you can see how the JSON response is represented inside an Appli array.
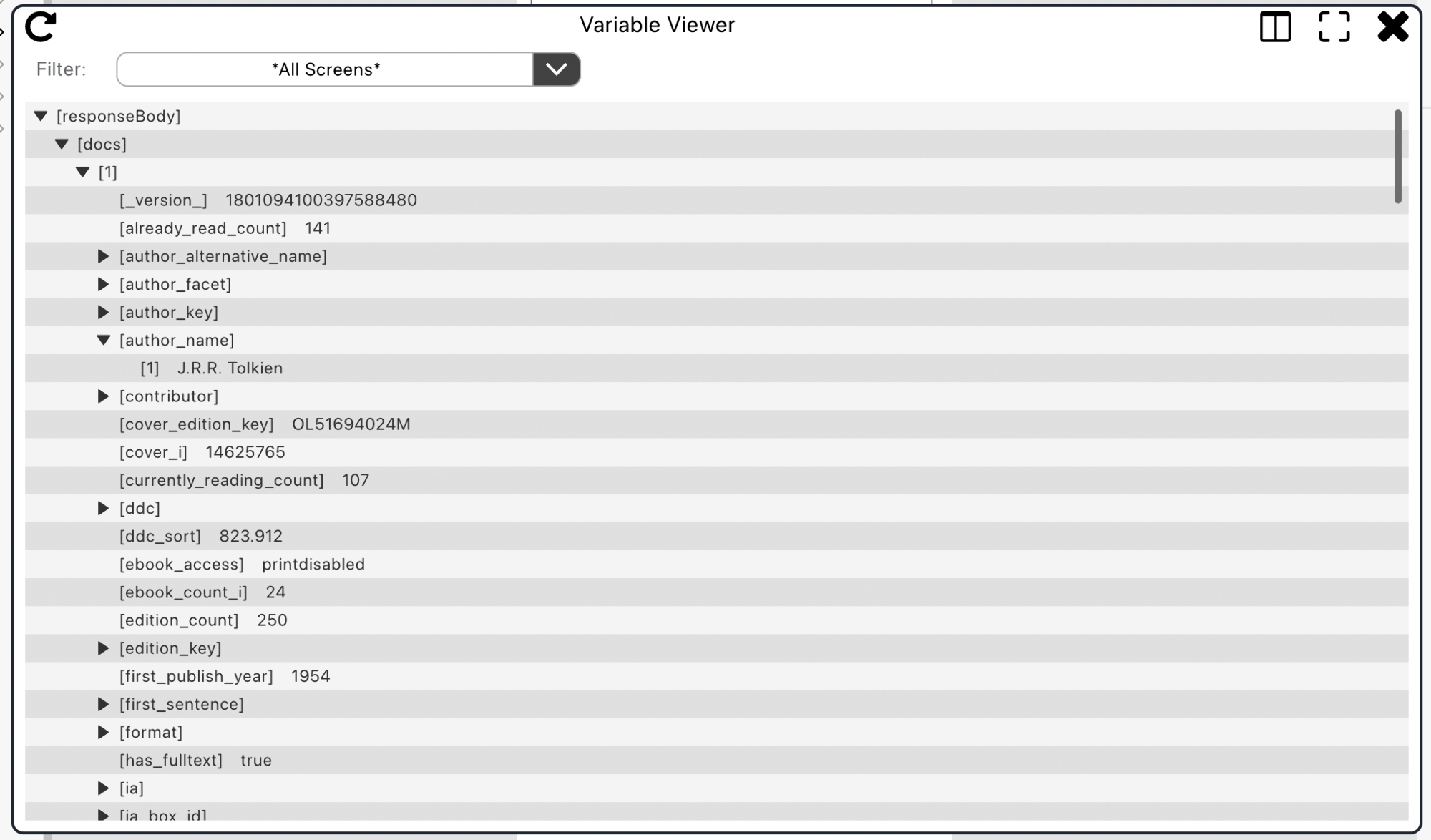
It is quite useful to execute partial scripts like this and then inspect the response using the variable viewer. It makes it easier to find out which array elements you need to assemble your user interface. Let’s complete the script now.
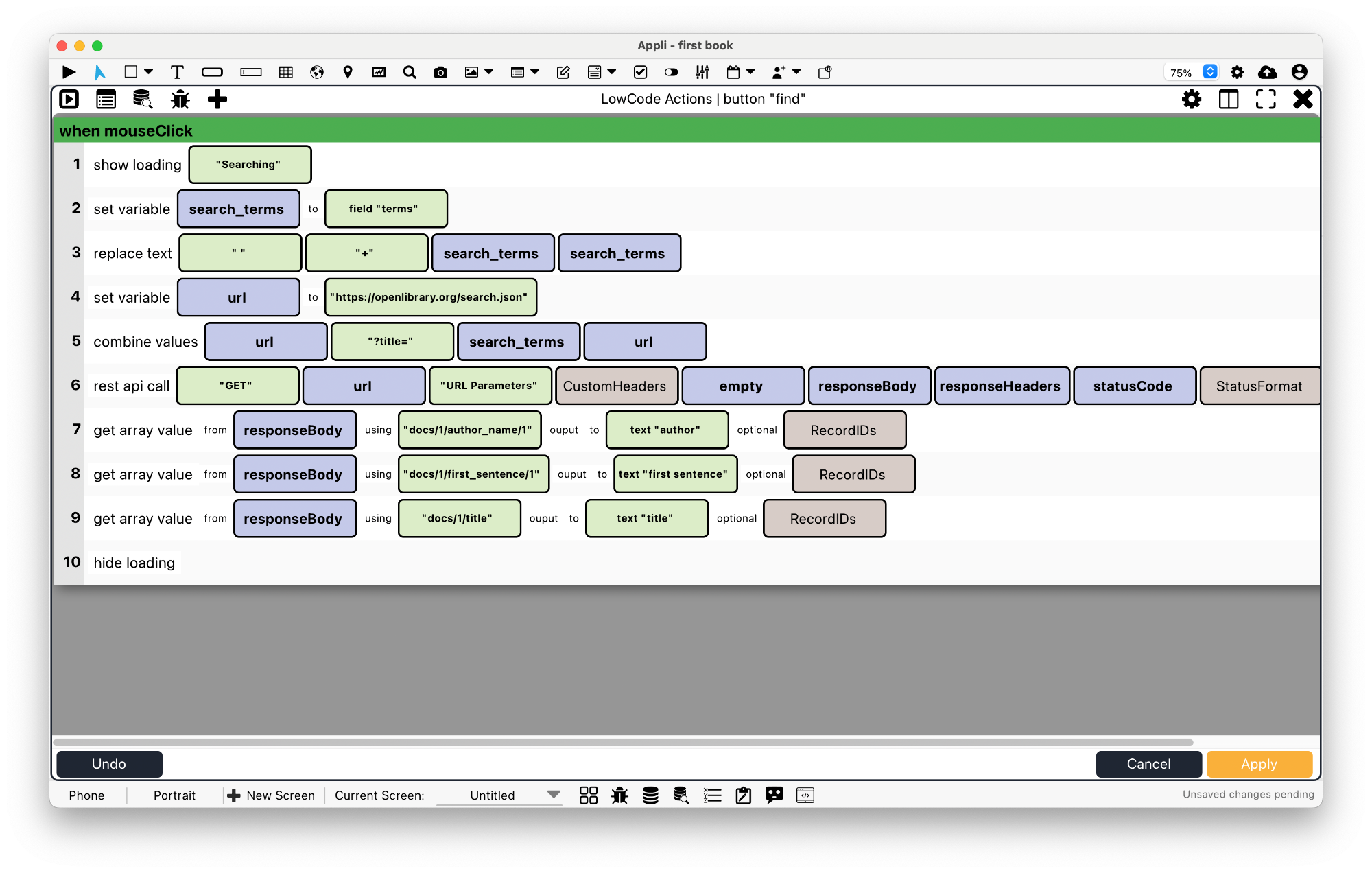
Check out the documentation for Get Array Value to learn more about how that action access deep array elements using path notation.
Using three Get Array Value actions, we can extract the title, author, and first sentence from the responseBody array and add them to the elements in the user interface.
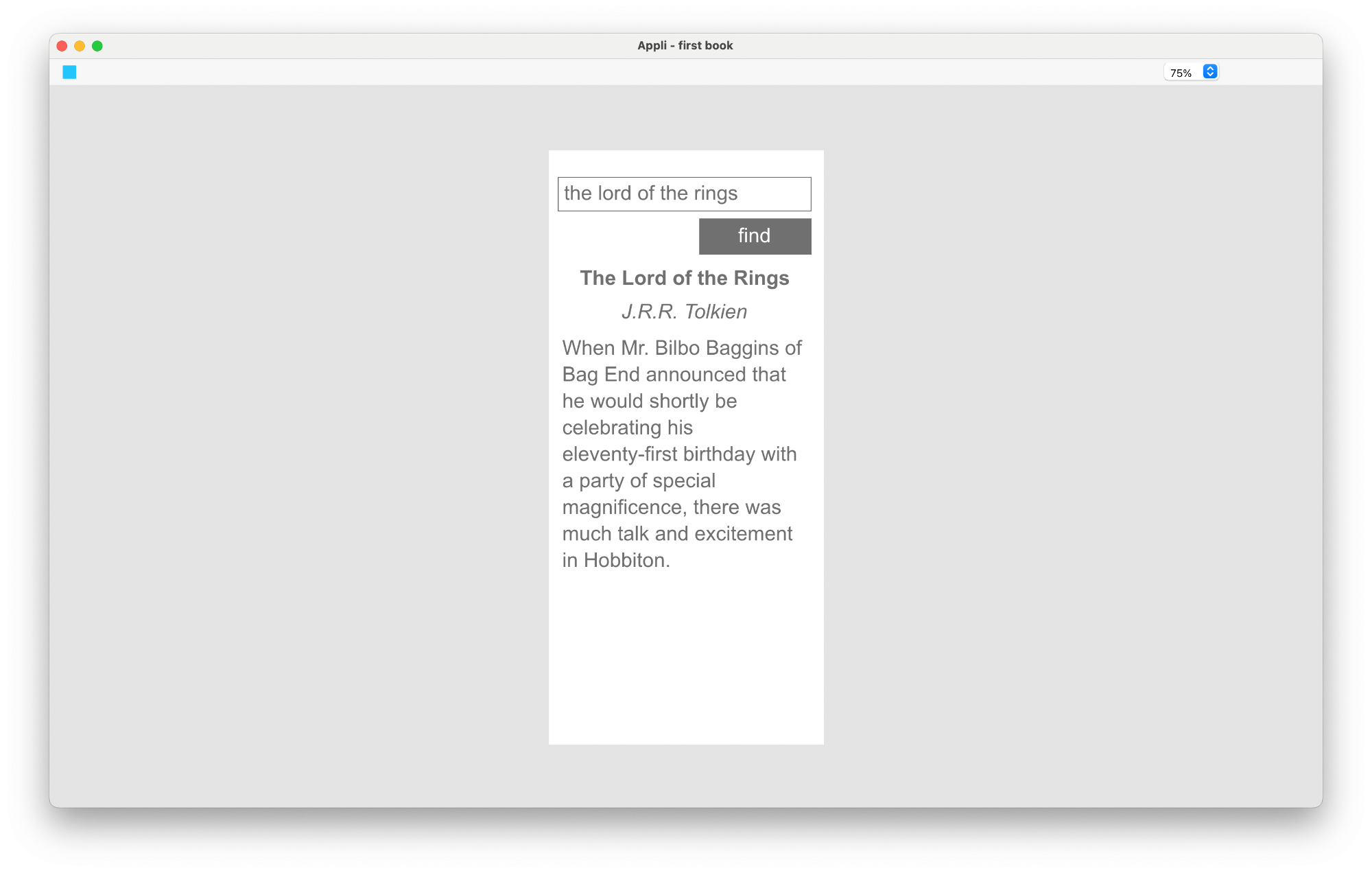
Final Words
This tutorial shows how to do a simple web request. To be an effective developer using Web API servers, you need to understand how Web Requests work. The best resource for this is The Web Mechanics section of MDN Webdocs. In there you’ll find how web requests work and how URLs are assembled and much more.
If you find yourself being challenged with some Web API server you want to interface with but doesn’t know exactly how to do it, reach out to us in our forum. Web Requests are an advanced topic and it is ok to ask for help when you need it.
This chapter was last updated on Tue 11 Jun 2024 17:27:35 BST